Python functions as dictionary values
Dec 21, 2015 • 2 minutes to read • Last Updated: Oct 29, 2017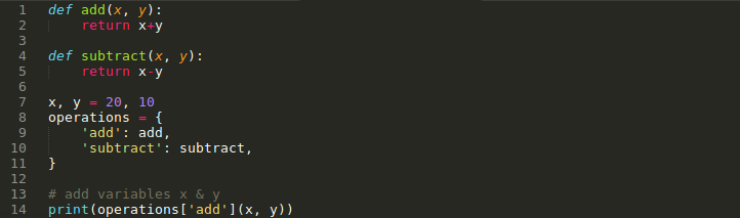
Pro pythonistas will tell you that you should actually be using python with higher order functions & as first class functions. Passing around functions are any programming languages greatest attribute. Here’s a trick to making function calls using python dictionaries.
Consider you have some functions add
, subtract
, divide
and multiply
. You could call these functions from a python dictionary like so:
def add(x, y):
return x+y
def subtract(x, y):
return x-y
def divide(x, y):
return x/y
def multiply(x, y):
return x*y
x, y = 20, 10
operations = {
'add': add,
'subtract': subtract,
'divide': divide,
'multiply': multiply,
}
# add variables x & y
print operations['add'](x, y)
# 30
# subtract variables x & y
print operations['subtract'](x, y)
# 10
# divide variables x & y
print operations['divide'](x, y)
# 2
# multiply variables x & y
print operations['multiply'](x, y)
# 100
To use variable size of arguments you could call the operations
dictionary with *args
def add(*args):
total = 0
for i in args:
total += i
return total
def subtract(x, y):
return x-y
x, y, z = 20, 10, 5
p, q, r = 30, 40, 50
operations = {
'add': add,
'subtract': subtract
}
# add variables x, y, z, p, q, r
print operations['add'](x, y, z, p, q, r)
# 155
# subtract variables x & y
print operations['subtract'](x, y)
# 10
I would love to hear what you think about this post, also know about your tricks to using python in the comments below.
I am writing a book!
While I do appreciate you reading my blog posts, I would like to draw your attention to another project of mine. I have slowly begun to write a book on how to build web scrapers with python. I go over topics on how to start with scrapy and end with building large scale automated scraping systems.
If you are looking to build web scrapers at scale or just receiving more anecdotes on python then please signup to the email list below.