Python's if __name__ == '__main__'
Kiran Koduru • Nov 20, 2017 • 2 minutes to read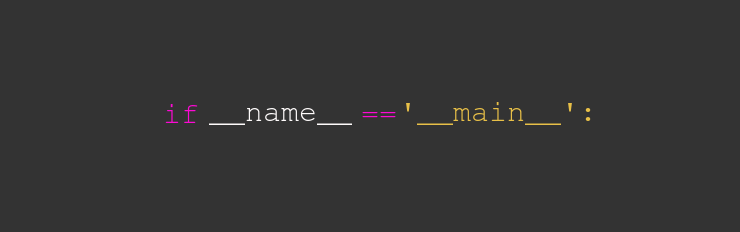
When you are new to python, you may have noticed that the variables that are widely used in all projects are __name__
in conjunction with __main__
.
They might be the most mentioned dunder variables in Python projects on github. To be specific, it is mentioned 6,436,149 times at the time of this writing.
__name__
__name__
is assigned to the module/file that it is associated with. It can also be assigned to module, classes, functions, methods, descriptors or generator instances.
# my_name_is_slim_shady.py
print(__name__) # will be set to `my_name_is_slim_shady`
class MyNameIsSlimShady(object):
def say_my_name(self):
print("My name is:")
Calling the my_name_is_slim_shady.py from a file what_is_my_name.py outputs my_name_is_slim_shady
and class name MyNameIsSlimShady
.
# what_is_my_name.py
print("What's my name?")
import my_name_is_slim_shady
# Outputs:
# What's my name?
# my_name_is_slim_shady
ss = my_name_is_slim_shady.MyNameIsSlimShady()
ss.say_my_name()
print(ss.__class__.__name__)
# Outputs:
# My name is:
# MyNameIsSlimShady
__main__
The __name__
variable for the module that is being executed is set to __main__
for a script, standard output or the interactive terminal. It is used to check if a module is invoked directly or called from a different file, see above description for __name__
.
When we run the file my_name_is_slim_shady.py alone we see that main_my_name_is_slim_shady()
function is invoked since the __name__
for the file is set to __main__
for this module.
# my_name_is_slim_shady.py
def main_my_name_is_slim_shady():
print("Standing Up!")
if __name__ == '__main__':
main_my_name_is_slim_shady()
# Output:
# Standing Up!
When I import the my_name_is_slim_shady.py file in what_is_my_name.py it doesn’t call the main_my_name_is_slim_shady()
function but invokes main_what_is_my_name()
.
# what_is_my_name.py
import my_name_is_slim_shady
def main_what_is_my_name():
print("Not Standing Up")
if __name__ == '__main__':
main_what_is_my_name()
# Output:
# Not Standing Up
This idea can be use for larger projects to set a starting point of execution for the whole project. Like in older versions of flask projects where you called app.run()
from a python file.
If you like reading this post, please do share or leave your comments on the post, I would love get more feedback on my articles. And if you really like my articles do subscribe to my newsletter. I promise to not spam.
I am writing a book!
While I do appreciate you reading my blog posts, I would like to draw your attention to another project of mine. I have slowly begun to write a book on how to build web scrapers with python. I go over topics on how to start with scrapy and end with building large scale automated scraping systems.
If you are looking to build web scrapers at scale or just receiving more anecdotes on python then please signup to the email list below.